Creating and Destroying Shapes
// Sample creation of a mesh shape in 'shape':
RED::Object* shape = RED::Factory::CreateInstance( CID_REDMeshShape );
// Sample destruction of a shape object 'shape':
RED::Object* resmgr = RED::Factory::CreateInstance( CID_REDResourceManager );
RED::IResourceManager* iresmgr = resmgr->As< RED::IResourceManager >();
RC_TEST( RED::Factory::DeleteInstance( shape, iresmgr->GetState() ) );
All HOOPS Luminate shapes are created from the RED::Factory::CreateInstance
method, using the appropriate class identifier. Shape class identifiers are defined below:
- Transformation shape:
CID_REDTransformShape
; See Transform Shapes for details- Triangle mesh shape:
CID_REDMeshShape
; See Mesh Shapes for details- Line shape:
CID_REDLineShape
; See Line Shapes for details- Point shape:
CID_REDPointShape
; See Point Shapes for details- Text shape:
CID_REDTextShape
; See Text Shapes for details- Volume shape:
CID_REDVolumeShape
; See Volumetric Shapes for details- Light source shape:
CID_REDLightShape
; See Light Shapes for details
The destruction of shapes is performed using RED::Factory::DeleteInstance
. The destruction of a shape is effective at the closing of the transaction used to perform the destruction order
It’s important to know that the destruction of a transform shape causes the destruction of all its children shape that are not linked to another shape that is not destroyed, as illustrated below:
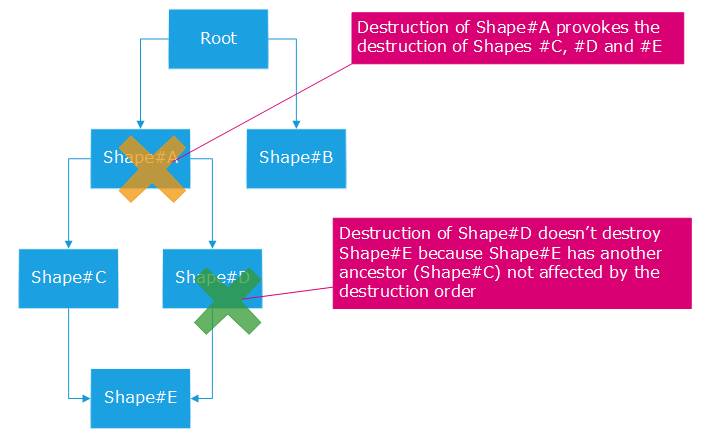
Scene graph destruction scenarios
In this example, The destruction of Shape#A provokes the destruction of its proprietary scene graph: this is the set of all recursive children of Shape#A that have no ancestor which is not a child of #A. In the example, this is composed of shapes #C, #D and #E. In the second destruction example, where Shape#D is destroyed, we see that only Shape#D will be destroyed. Shape#E is NOT included in the scope of the destruction because Shape#E is child of Shape#C and Shape#C is NOT destroyed.
These behaviors have to be considered to avoid doing duplicate destruction of shapes. If a shape is deleted twice using RED::Factory::DeleteInstance
(either directly, or after a shape tree destruction), RED_SCG_SHAPE_ALREADY_DESTROYED
will be returned.
Another important point is that the destruction of a viewpoint causes the destruction of the root shape of the viewpoint (see RED::IViewpoint::GetRootShape
), and consequently of all the scene graph below that viewpoint, unless there are parent links to other scene graphs.