Generic Material
Introduction
HOOPS Luminate comes with a lot of built-in shaders to quickly assemble all kind of materials. It also provides ready-to-use materials with nice features. The generic material is one of them.
Description
The generic material is intended for good quality/speed ratio visualization. It features what most people would expect from a all-purpose material. See Using the Generic Material for details.
In this tutorial, we create three spheres each one receiving a different generic material. Because of the number of generic material parameters being quite high, HOOPS Luminate provides additional helper methods to create subsets of generic materials. First, we create a purely diffuse texture-based material:
// Diffuse material.
RED::Object* texture = NULL;
RC_TEST( iresmgr->CreateImage2D( texture, iresmgr->GetState() ) );
RC_TEST( RED::ImageTools::Load( texture, "../resources/wood.png", RED::FMT_RGB, false, false, RED::TGT_TEX_2D, iresmgr->GetState() ) );
RC_TEST( imaterial->SetupGenericDiffuseMaterial( false,
RED::Color::BLACK, texture, RED::Matrix::IDENTITY, RED::MCL_TEX0,
&g_ls_real_time, &g_ls_photo,
resmgr, iresmgr->GetState() ) );
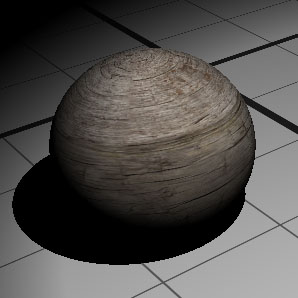
Each generic material can be defined for one or two configurations: hardware, software or both. It means that the resulting material embeds two lists of shaders tailored specifically for the graphics hardware of the software rendering, giving the best of each world. Based on the current rendering mode, the user can then choose which configuration must be used (this is achieved through the layer set mechanism of HOOPS Luminate applied to viewpoints)!
Then, a bumpy diffuse material is created:
// Diffuse material with bump.
RED::Object* bump = NULL, *texture = NULL;
RC_TEST( iresmgr->CreateImage2D( texture, iresmgr->GetState() ) );
RC_TEST( RED::ImageTools::Load( texture, "../resources/marble.png", RED::FMT_RGB, false, false, RED::TGT_TEX_2D, iresmgr->GetState() ) );
// Bump textures are normal maps in RED. So, we convert the texture to a valid
// normal map before using it as a bump texture.
RC_TEST( iresmgr->CreateImage2D( bump, iresmgr->GetState() ) );
RC_TEST( bump->As< RED::IImage2D >()->NormalMap( RED::FMT_RGB, RED::TGT_TEX_2D, 3.0f, texture, iresmgr->GetState() ) );
// Tangents are needed to apply bump on meshes.
RC_TEST( isphere->BuildTangents( RED::MCL_USER0, RED::MCL_TEX0, iresmgr->GetState() ) );
RC_TEST( imaterial->SetupGenericBumpyDiffuseMaterial( false,
RED::Color::BLACK, texture, RED::Matrix::IDENTITY, RED::MCL_TEX0,
bump, RED::Matrix::IDENTITY, RED::MCL_TEX0, RED::MCL_USER0,
&g_ls_real_time, &g_ls_photo,
resmgr, iresmgr->GetState() ) );
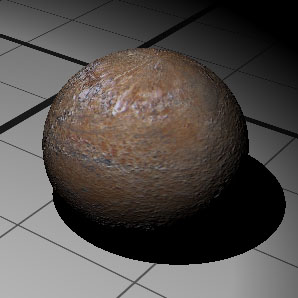
Geometries using bump maps need tangent vectors. Starting from valid texture coordinates, the RED::IMeshShape::BuildTangents
method computes tangent vectors for you.
Finally, a more complex material is created: a Fresnel glass. It’s a material which is both reflective and refractive. The amount of each effect smoothly varies with the light incoming angle. Here, we use automatic environment maps for reflections in real-time as it fits perfectly the shape of the sphere:
// Fresnel refractive material.
RC_TEST( imaterial->SetupGenericMaterial( true, true,
RED::Color::BLACK, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0,
RED::Color::BLACK, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0,
RED::Color::BLACK, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0,
RED::Color::BLACK, NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, 0.f,
RED::Color( 0.95f ), NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, 0.f,
true, false, NULL, RED::Matrix::IDENTITY,
4.0f, true, RED::Color( 0.05f ), NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, 0.f,
NULL, RED::Matrix::IDENTITY, RED::MCL_TEX0, RED::MCL_TEX0,
&g_ls_real_time, &g_ls_photo,
resmgr, iresmgr->GetState() ) );
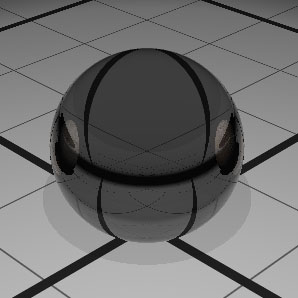