HOOPS Luminate Scene Graph Overview
This book details the scene graph part of the HOOPS Luminate API. The scene graph is a key element in the definition of a 2D or 3D world to display. During the course of this book, we’ll review all the different objects that are part of the HOOPS Luminate scene graph and see how they can be organized together.
HOOPS Luminate scene graph objects are divided into two categories:
- Shapes: these are the graphic entities to display. Shapes can be composed of all common graphical entities: triangles, lines, points, texts, lights, …
- Atomic attributes: these define the way a given scene graph is to be displayed on screen. Among them, we’ll find bounding spheres, layersets, matrices, …
What is a Scene Graph?
The HOOPS Luminate scene graph is a graph of shapes. More precisely, it’s a Direct Acyclic Graph. We’ll often refer to this using the “DAG” acronym. A direct acyclic graph defines:
- A parent to children relationship among its shapes
- It don’t support loops. Consequently, the graph has a root element and leaves elements
- A reference / instance relationship: a given shape may have more than one parent
The picture below illustrates these properties:
We identify An instanced shape is stored once in memory (the reference) and is visualized as many times as there are different paths from the scene graph root down to the shape itself (the instances).
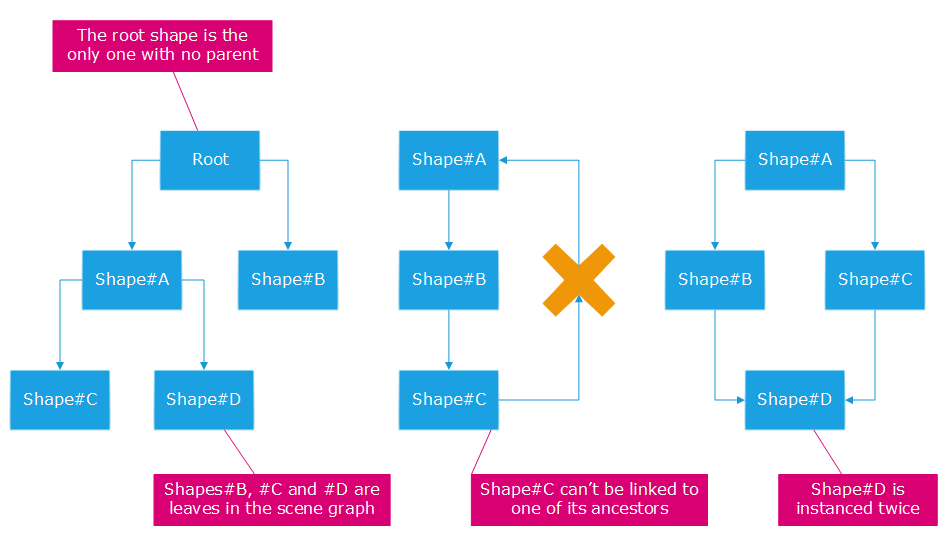
The Direct Acyclic Graph (DAG) properties.
Creating and Destroying Shapes
// Sample creation of a mesh shape in 'shape':
RED::Object* shape = RED::Factory::CreateInstance( CID_REDMeshShape );
// Sample destruction of a shape object 'shape':
RED::Object* resmgr = RED::Factory::CreateInstance( CID_REDResourceManager );
RED::IResourceManager* iresmgr = resmgr->As< RED::IResourceManager >();
RC_TEST( RED::Factory::DeleteInstance( shape, iresmgr->GetState() ) );
All HOOPS Luminate shapes are created from the RED::Factory::CreateInstance
method, using the appropriate class identifier. Shape class identifiers are defined below:
- Transformation shape:
CID_REDTransformShape
; See Transform Shapes for details- Triangle mesh shape:
CID_REDMeshShape
; See Mesh Shapes for details- Line shape:
CID_REDLineShape
; See Line Shapes for details- Point shape:
CID_REDPointShape
; See Point Shapes for details- Text shape:
CID_REDTextShape
; See Text Shapes for details- Volume shape:
CID_REDVolumeShape
; See Volumetric Shapes for details- Light source shape:
CID_REDLightShape
; See Light Shapes for details
The destruction of shapes is performed using RED::Factory::DeleteInstance
. The destruction of a shape is effective at the closing of the transaction used to perform the destruction order
It’s important to know that the destruction of a transform shape causes the destruction of all its children shape that are not linked to another shape that is not destroyed, as illustrated below:
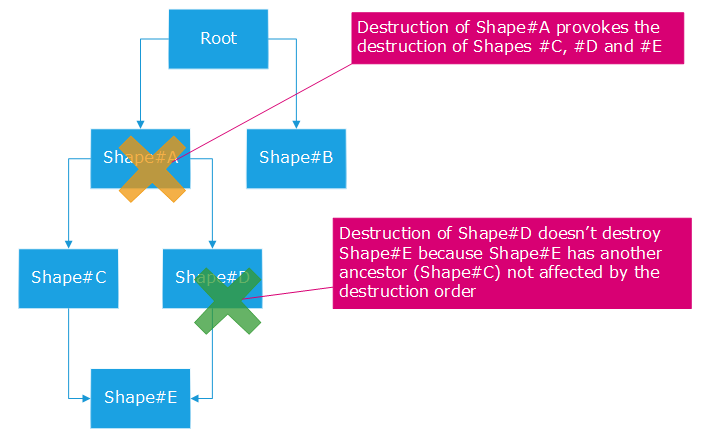
Scene graph destruction scenarios
In this example, The destruction of Shape#A provokes the destruction of its proprietary scene graph: this is the set of all recursive children of Shape#A that have no ancestor which is not a child of #A. In the example, this is composed of shapes #C, #D and #E. In the second destruction example, where Shape#D is destroyed, we see that only Shape#D will be destroyed. Shape#E is NOT included in the scope of the destruction because Shape#E is child of Shape#C and Shape#C is NOT destroyed.
These behaviors have to be considered to avoid doing duplicate destruction of shapes. If a shape is deleted twice using RED::Factory::DeleteInstance
(either directly, or after a shape tree destruction), RED_SCG_SHAPE_ALREADY_DESTROYED
will be returned.
Another important point is that the destruction of a viewpoint causes the destruction of the root shape of the viewpoint (see RED::IViewpoint::GetRootShape
), and consequently of all the scene graph below that viewpoint, unless there are parent links to other scene graphs.
Shapes Types in a Scene Graph
The HOOPS Luminate scene graph features the following base shape types that can be used to assemble the visible geometrical primitives of a scene to render:
Shape Name | CID | Main Interfaces | Illustrations |
---|---|---|---|
Mesh Shapes | CID_REDMeshShape |
|
![]() |
Line Shapes | CID_REDLineShape |
|
![]() |
Point Shapes | CID_REDPointShape |
|
![]() |
Transform Shapes | CID_REDTransformShape |
|
![]() |
Text Shapes | CID_REDTextShape |
|
![]() |
Light Shapes | CID_REDLightShape |
|
![]() |
Volumetric Shapes | CID_REDVolumeShape |
|
![]() |
HOOPS Luminate’s mesh, line and point display shapes are just atomic. They respectively load triangles, line segments (or strips) and points. There’s no built-in tessellation service HOOPS Luminate except for planar polygons. HOOPS Luminate data is usually sourced from external modelling software that provide the data arrays to use to fill-in the shapes. Each shape is a RED::Object
created by RED::Factory::CreateInstance
using the appropriate CID and implementing the following interfaces:
There are also several other shape types available in HOOPS Luminate:
Transformation shapes are mandatory to define the relative placement of objects. They actually define the real positioning of objects thanks to the transformation matrix they store. Texts are 2D or 3D primitives that are used to display various types of texts inside an application. Light sources are needed to provide some illumination to the geometries found inside a scene graph, and volumetric effects are rather advanced objects used for the definition of gaseous effects in a high quality image rendering.
Scene Graph Attributes
A shape stores several attributes that indicate HOOPS Luminate how to display the shape, or how external application data can be linked to a shape. Attributes are standalone or may be inherited along the hierarchy defined by the scene graph DAG. The details of these attributes and how they are to be used is here: Shape Attributes in a Scene Graph.
Culling
HOOPS Luminate delivers a number of built-in culling methods that can be used to speed-up the display of some scenes. All these methods are reviewed in the paragraph here: Culling Methods