Creating an Instanced Shape
An instanced shape is a shape that exists once in the scene graph and that is visualized several times. We’ll assemble the following scene graph in this task example:
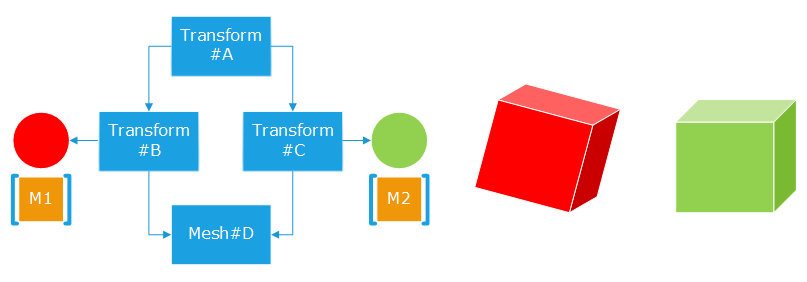
Mesh#D is instanced twice. It has two direct parents: Transform#B and Transform#C. Therefore, if we parse the scene graph from Transform#A, we have two shapes to visualize: The first shape instance has path #ABD and the second one has #ACD. At the Transforms #B and #C level, we see that we have different materials and transformation matrices. This can be used to make each instance unique.
The code needed to construct this assembly is:
// Create our shapes and access their interfaces:
RED::Object* shapeA = RED::Factory::CreateInstance( CID_REDTransformShape );
RED::ITransformShape* ishapeA = shapeA->As< RED::ITransformShape >();
RED::Object* shapeB = RED::Factory::CreateInstance( CID_REDTransformShape );
RED::ITransformShape* ishapeB = shapeB->As< RED::ITransformShape >();
RED::Object* shapeC = RED::Factory::CreateInstance( CID_REDTransformShape );
RED::ITransformShape* ishapeC = shapeC->As< RED::ITransformShape >();
RED::Object* shapeD = RED::Factory::CreateInstance( CID_REDMeshShape );
// Assemble the scene graph:
RC_TEST( ishapeA->AddChild( shapeB, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
RC_TEST( ishapeA->AddChild( shapeC, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
RC_TEST( ishapeB->AddChild( shapeD, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
RC_TEST( ishapeC->AddChild( shapeD, RED_SHP_DAG_NO_UPDATE, iresmgr->GetState() ) );
Then, from this code, you simply need to setup matrices, materials, layersets or any other shape attribute to distinguish the two instances of Mesh#D. See Shape Attributes in a Scene Graph for details.