Building a Geometry - Part 3 - Adding Lights
This tutorial will explain how to setup the lights contained in a geometry.
In the “Building a Geometry - Part 1 - Loading a Source Scene” tutorial, we saw that when we load a geometry, the lights contained in the original scene graph are imported.
The ART::IAssetManager::LoadGeometrySource
method automatically builds ART::ILight
objects and set them in the geometry. We can get them: ART::IGeometry::GetLightsCount
and ART::IGeometry::GetLight
.
// Getting the lights and parameterize them.
ART::IGeometry* igeometry = g_geometry->As< ART::IGeometry >();
int count = igeometry->GetLightsCount();
RED::Object* light;
ART::ILight* ilight;
for( int i = 0; i < count; ++i )
{
light = igeometry->GetLight( i );
ilight = light->As< ART::ILight >();
// Lights are deactivated by default.
RC_TEST( ilight->SetActive( true ) );
// Setting the baking mode corresponding to the GI cache content.
if( i == 0 )
{
ilight->SetBaking( ART::LB_FULL );
}
else if( i == 1 )
{
ilight->SetBaking( ART::LB_INDIRECT );
}
else
{
ilight->SetBaking( ART::LB_NONE );
}
// Other parameters can be overridden here.
// If not, the parameters are those from the original file.
}
The ART::ILight
interface contain several accessors to setup the multiple light parameters (you can go to the ART::ILight
documentation for a complete listing of the methods):
- active / not active
- shadows
- spot angle
- color
- IES profile
- etc.
By default, all the lights are deactivated. We must enable them if we want to see something.
The light baking mode must be set accordingly to the GI cache content. If a light has been baked (full or indirect only), ART::ILight::SetBaking
must be called with the corresponding values. Baked lights can’t be moved in the scene once precalculated. However they can be activated and deactivated. Dynamic lights (ART::LB_NONE
) can be moved and are updated every frame (position, orientation, shadows, etc.). Currently, only spot and point lights can be dynamic. All other RED lights must be baked.
The shadowing can be only be activated on the dynamic spot lights using shadow maps. There are several options:
ART::ILight::SetShadowMap
ART::ILight::SetShadowMapSize
ART::ILight::SetShadowBias
ART::ILight::SetStaticShadowMap
- allows to compute the shadow map only when necessary contrary to every frame - for performance
The REDart lights support IES profiles. The IES data are loaded with the geometry lights if they are in the original file or they can be added later using ART::IAssetManager::LoadIESFile
and ART::ILight::SetIESData
.
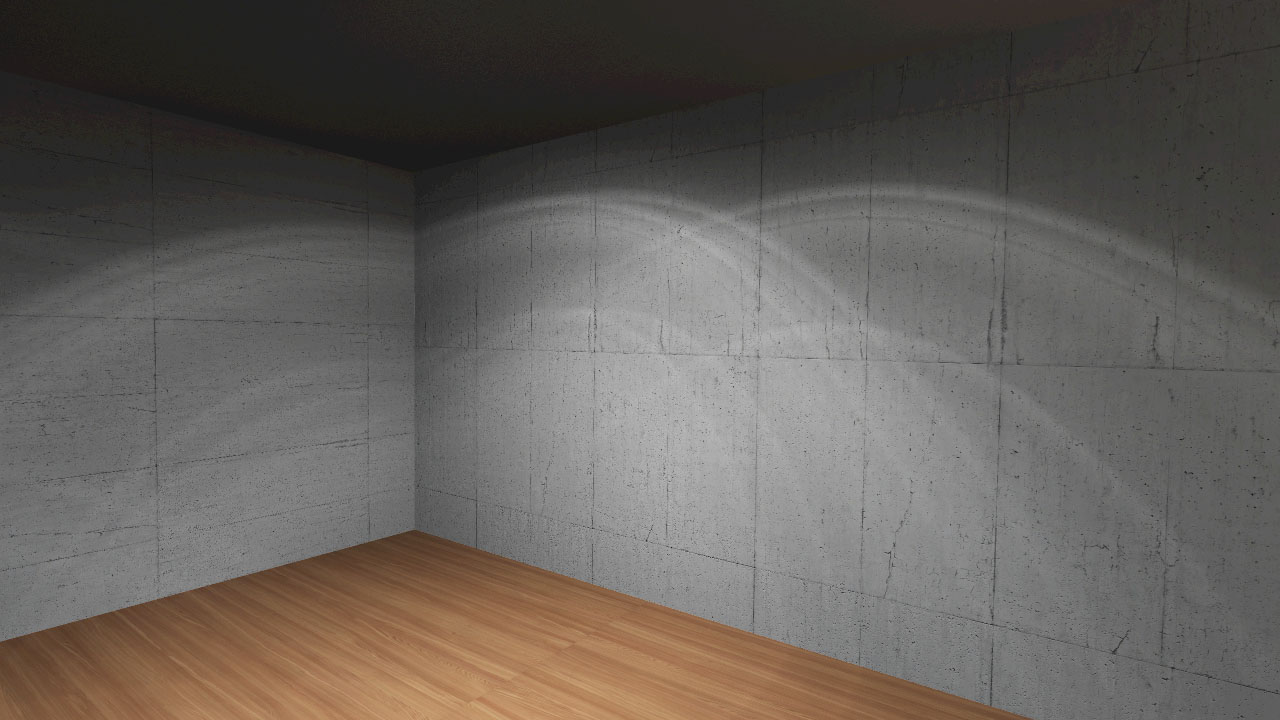
IES light in the house (only indirect is baked to keep the clear IES profile)