Setup a Rectangular Area Light
A rectangular area light is a surfacic light that has a rectangular shape. It works both on the CPU and the GPU and renders quite fast on the GPU on almost any graphic hardware, which makes it very useful for all pre-visualization needs.
Like all the lights, the first thing to do is to create it:
// Create the light shape:
RED::Object* light = RED::Factory::CreateInstance( CID_REDLightShape );
if( light == NULL )
RC_TEST( RED_ALLOC_FAILURE );
RED::ILightShape* ilight = light->As< RED::ILightShape >();
Then we have to define it as a rectangular area light:
// The rectangular area light parameters:
RED::Vector3 position = RED::Vector3( 20.0, 20.0, 60.0 );
RED::Vector3 direction = RED::Vector3::ZERO - position;
RED::Vector3 up = RED::Vector3::ZAXIS;
float intensity = 500.0f;
RED::Color diffuse = RED::Color::WHITE;
RED::Color specular = RED::Color::WHITE;
int samples_count = 128;
float width = 100.0f;
float height = 50.0f;
// Define the light as a rectangular area light:
RC_TEST( ilight->SetRectangularAreaLight( intensity, position, direction, up, diffuse, specular, samples_count, width, height, iresmgr->GetState() ) );
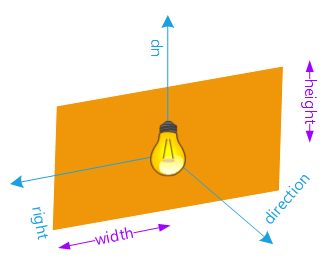
Rectangular area light parameters
Note that the rectangular area light always has a quadratic decay that result of the visible solid angle of the light from the illuminated point.
And finally, we have to add it to the viewpoint:
// Add the light to the viewpoint:
RC_TEST( icamera->AddShape( light, iresmgr->GetState() ) );