Programming Language
HOOPS Luminate is a C++ API. Therefore, HOOPS Luminate gets used after inclusion of header files that are all located in the ‘HOOPS Luminate.m’ module of HOOPS Luminate under the product installation filetree:
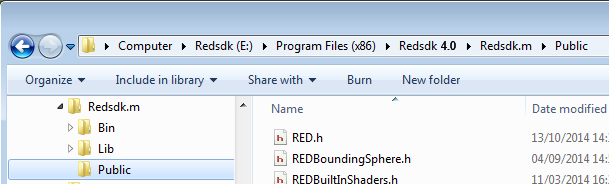
Location of the HOOPS Luminate 4.0 header files under the installation filetree
There are two categories of objects in HOOPS Luminate:
Atomic classes, such as:
RED::Matrix
,RED::StateShader
orRED::String
Interfaces, such as
RED::IShape
,RED::ISoftLight
orRED::IDataManager
Atomic classes are generally used a method parameters and can generally be created on the stack and destroyed after use. For example:
// Example of a matrix created locally to the method, and used in a method:
{
RED::Matrix matrix;
matrix.SetTranslation( RED::Vector3( 1.0f, 10.0f, 100.0f ) );
// As an example, set the matrix as a transform node pivot matrix. 'itransform' is a RED::ITransformShape
// interface, 'iresmgr' is our cluster's resource manager interface:
RC_TEST( itransform->SetPivotAxis( matrix, iresmgr->GetState() ) );
// On exiting this code block, 'matrix' is released from memory.
}
Interfaces are accessed from HOOPS Luminate objects. Many API methods are manipulating RED::Object
pointers. These objects are often returned by HOOPS Luminate creation methods (such as the RED::Factory::CreateInstance
call). Given the object, it generally implements a set of services that can be accessed through interfaces:
Accessing to RED Object Interfaces
From the user point of view, all the RED objects created through the RED::Factory
and passed to the HOOPS Luminate API are of the class RED::Object
. This base class implements a very small set of methods which mainly consists of the dynamic casting mechanism. The casting mechanism enables the user to retrieve sets of methods, called interfaces, from those base objects.
Hence, here is the example of the creation of a viewpoint:
RED::Object* viewpoint = RED::Factory::CreateInstance( CID_REDViewpoint );
RED::IViewpoint* iviewpoint = viewpoint->As< RED::IViewpoint >();
The list of valid CIDs can be found in REDCID.h
. Another example with a light source:
RED::Object* light = RED::Factory::CreateInstance( CID_REDLightShape );
RED::ILightShape* ilight = light->As< RED::ILightShape >();
Some objects may implement several interfaces. For example, the light source created above also implements the RED::IShape
interface:
RED::IShape* ishape = light->As< RED::IShape >();
These interfaces allow a smooth evolution of the HOOPS Luminate services at a minimal change cost for the calling applications over the successive API versions.
Cross Platform Compilation
HOOPS Luminate builds on Windows x64; Linux x64 and MacOSX x64. Some #define statements are required to ensure a proper compilation of HOOPS Luminate under each platform:
_WIN32 and _WIN64 on Windows x64
_LIN32 and _LIN64 on Linux x64
_MAC32 on Mac OSX x64
Please make sure that the right set of #define values are activated for each platform. If these statements are not properly defines, HOOPS Luminate may compile in your software, but run-time errors will probably crash your application, due to incorrect virtual tables or wrong objects byte sizes.
Linking with HOOPS Luminate
To link HOOPS Luminate with an application, please refer to the Deployment page.
Coding Principles
The HOOPS Luminate API is quite small and compact. This is by design choice. We have tried to follow a few rules in the definition of the API and in the names of API parameters:
Method input parameters have names starting with a lowercase ‘i’ letter
Method output parameters have names starting with a lowercase ‘o’ letter
Generally speaking, output parameters come first in the method’s parameters, before input parameters
RED_RC MyMethod( RED::Object*& oParameterOutput, unsigned int iParameterInput );
Most SDK methods do return a
RED_RC
return code value: HOOPS Luminate does not use any C++ exception, not to interfere with the hosting application exception management policy. CheckingRED_RC
returned values is vital for any HOOPS Luminate application. There are many return codes, and ensuring that applications don’t raise unexpected return codes is a mandatory step for a proper HOOPS Luminate usage.
API Evolutions
The HOOPS Luminate API is not frozen. This means that each new version of HOOPS Luminate will deliver new APIs needed to use the new features that were implemented by the Tech Soft 3D team. Besides that, the Tech Soft 3D team may change existing APIs over the time. This may require some changes in the HOOPS Luminate client applications. Our objective is to keep the HOOPS Luminate API clear and usable to all. Therefore, there’s no API deprecation in HOOPS Luminate: we do remove a method if it has become useless or if it’s replaced by a newer more efficient other service.
In all cases, we publish a HOOPS Luminate migration guide to follow to successfully migrate from a HOOPS Luminate version to another one.